Introduction
Python is a versatile programming language that offers various tools and features to make coding more efficient and organized. One such feature is the classmethod() function, which allows us to define methods that are bound to the class rather than an instance of the class. In this article, we will explore the concept of classmethod() in Python, its benefits, syntax, usage, differences from staticmethod(), implementation examples, best practices, and common mistakes to avoid.
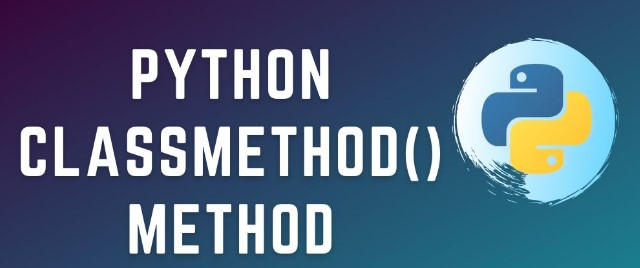
Learn Introduction to Python Programming. Click here.
Table of contents
What is a classmethod() in Python?
A classmethod() is a built-in function in Python that is used to define a method that is bound to the class and not the instance of the class. It is denoted by the @classmethod decorator and can be accessed directly from the class itself, without the need for creating an instance of the class.
Using classmethod() offers several benefits in Python programming. Firstly, it allows us to define methods that can be accessed directly from the class, making the code more readable and organized. Secondly, classmethod() provides a way to modify class attributes, which can be useful in certain scenarios. Lastly, classmethod() enables us to implement inheritance more efficiently, as we will explore later in this guide.
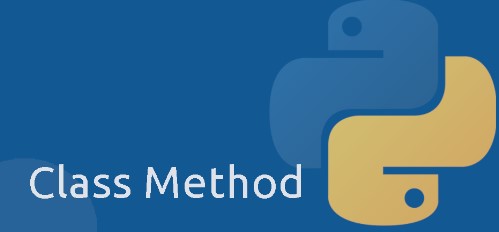
Syntax and Usage of classmethod()
The syntax for defining a classmethod() is as follows:
class MyClass:
@classmethod
def my_method(cls, arg1, arg2, ...):
# method implementation
In the above syntax, `my_method` is the name of the class method, and `arg1`, `arg2`, … are the arguments that the method can accept. The `cls` parameter is automatically passed to the method and refers to the class itself.
To use a classmethod(), we can directly call it from the class, without the need for creating an instance of the class. For example:
MyClass.my_method(arg1, arg2, ...)
Differences between classmethod() and staticmethod()
Although both classmethod() and staticmethod() are used to define methods that are bound to the class rather than an instance, there are some key differences between them.
- The main difference lies in the way they handle the first parameter. In classmethod(), the first parameter is automatically passed and refers to the class itself (usually named `cls`), whereas in staticmethod(), no parameters are automatically passed.
- Another difference is that classmethod() can access and modify class attributes, whereas staticmethod() cannot. This makes classmethod() more suitable for scenarios where we need to work with class-level data.
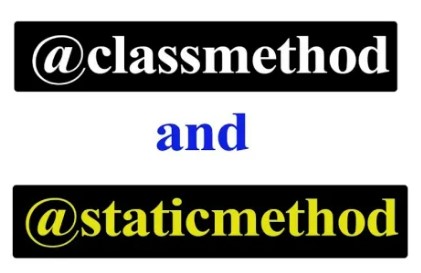
Examples of classmethod() Implementation
Let’s explore some examples to understand how classmethod() can be implemented in Python.
Creating Class Methods
class Circle:
pi = 3.14159
@classmethod
def calculate_area(cls, radius):
return cls.pi * radius * radius
# Calling the class method
area = Circle.calculate_area(5)
print("Area of the circle:", area)
In the above example, we define a class method `calculate_area()` in the `Circle` class. This method calculates the area of a circle using the class attribute `pi` and the given radius. We can directly call the class method using the class name, without creating an instance of the class.
Accessing Class Attributes and Methods
class Rectangle:
length = 0
width = 0
def __init__(self, length, width):
self.length = length
self.width = width
@classmethod
def create_square(cls, side):
return cls(side, side)
# Creating a square using the class method
square = Rectangle.create_square(5)
print("Square length:", square.length)
print("Square width:", square.width)
In this example, we define a class method `create_square()` in the `Rectangle` class. This method creates a square by initializing the length and width with the same value. We can access and modify the class attributes `length` and `width` using the class method.
Modifying Class Attributes
class Counter:
count = 0
def __init__(self):
Counter.count += 1
@classmethod
def get_count(cls):
return cls.count
# Creating instances of the class
c1 = Counter()
c2 = Counter()
c3 = Counter()
# Accessing the class attribute using the class method
print("Count:", Counter.get_count())
In this example, we define a class method `get_count()` in the `Counter` class. This method returns the value of the class attribute `count`, which keeps track of the number of instances created. We can access the class attribute using the class method, without the need for creating an instance.
Inheritance and classmethod()
class Animal:
legs = 4
@classmethod
def get_legs(cls):
return cls.legs
class Dog(Animal):
breed = "Labrador"
@classmethod
def get_breed(cls):
return cls.breed
# Accessing class attributes and methods through inheritance
print("Number of legs:", Dog.get_legs())
print("Breed:", Dog.get_breed())
In this example, we define a class method `get_legs()` in the `Animal` class, which returns the number of legs. The `Dog` class inherits from the `Animal` class and defines its own class method `get_breed()`, which returns the breed of the dog. We can access both the class attributes and methods through inheritance.
Future Consideration: Using __class__
For readers seeking a deeper understanding, an alternative to the cls parameter is the use of the __class__ attribute. While cls is conventionally used and recommended, understanding __class__ can provide insights into the inner workings of Python.
The __class__ attribute refers to the class of an instance. When used within a class method, it represents the class itself. While this approach is more advanced and not commonly used in everyday scenarios, exploring it can deepen your grasp of Python’s mechanisms.
class Example:
data = "Example class"
@classmethod
def display_class_name(cls):
print("Class name:", cls.__name__)
print("Using __class__ attribute:", cls.__class__.__name__)
# Calling the class method
Example.display_class_name()
In this example, cls.__name__ and cls.__class__.__name__ both yield the class name. While cls.__name__ directly accesses the class name, cls.__class__.__name__ accesses the class name via the __class__ attribute.
Keep in mind that using __class__ directly is less conventional and may be unnecessary in typical use cases. However, it can be a valuable exploration for those interested in delving deeper into the Python language.
When to Use classmethod() in Python
classmethod() is useful in various scenarios, such as:
- When we need to define methods that are bound to the class and not the instance.
- When we want to access or modify class attributes.
- When implementing inheritance and need to work with class-level data.
By using classmethod(), we can make our code more organized, readable, and efficient.
Common Mistakes and Pitfalls with classmethod()
While using classmethod(), there are some common mistakes and pitfalls to avoid:
- Forgetting to use the @classmethod decorator before defining the method.
- Not passing the `cls` parameter in the method definition.
- Accidentally using staticmethod() instead of classmethod() or vice versa.
- Modifying mutable class attributes directly without using the class method.
By being aware of these mistakes, we can ensure the proper usage of classmethod() in our code.
Conclusion
In this article, we explored the concept of classmethod() in Python. We learned about its benefits, syntax, usage, differences from staticmethod(), and various examples of its implementation. We also discussed when to use classmethod(), and common mistakes to avoid. By understanding and utilizing classmethod() effectively, we can enhance our Python programming skills and create more organized and efficient code.
You can also refer to these articles to know more:
Frequently Asked Questions
A1: The main purpose of using classmethod() in Python is to define methods that are bound to the class rather than an instance of the class. It allows direct access from the class itself without the need to create an instance. classmethod() is particularly useful when working with class-level data, accessing or modifying class attributes, and implementing inheritance efficiently.
A2: The key difference between classmethod() and staticmethod() lies in how they handle the first parameter. In classmethod(), the first parameter is automatically passed and refers to the class itself (usually named cls
), while staticmethod() does not automatically pass any parameters. Another distinction is that classmethod() can access and modify class attributes, making it suitable for scenarios where class-level data manipulation is required.
A3: No, classmethod() is not typically used for creating instances of a class. Its primary purpose is to define methods that operate on the class itself rather than instances. For creating instances, the standard constructor method __init__
is more appropriate.
A4: classmethod() is beneficial in inheritance as it allows child classes to access and utilize class-level methods and attributes defined in the parent class. This facilitates a more efficient and organized implementation of inheritance, enabling child classes to inherit and extend functionality from the parent class through class methods.
Related
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/01/understanding-classmethod-in-python/
- :is
- :not
- :where
- 1
- 11
- 12
- 13
- a
- About
- above
- Accept
- access
- accessed
- accessing
- advanced
- allows
- also
- alternative
- an
- and
- animal
- Another
- any
- approach
- appropriate
- ARE
- AREA
- arguments
- article
- articles
- AS
- attributes
- automatically
- avoid
- aware
- BE
- before
- being
- beneficial
- benefits
- BEST
- best practices
- between
- both
- bound
- BREED
- built-in
- by
- calculates
- call
- calling
- CAN
- cannot
- cases
- certain
- child
- Circle
- class
- classes
- click
- code
- Coding
- Common
- commonly
- concept
- consideration
- conventional
- count
- Counter
- create
- created
- creates
- Creating
- data
- Deepen
- deeper
- define
- defined
- Defines
- defining
- definition
- differ
- difference
- differences
- direct
- Direct access
- directly
- discussed
- distinction
- does
- Dog
- effectively
- efficient
- efficiently
- enables
- enabling
- enhance
- ensure
- Ether (ETH)
- everyday
- example
- examples
- exploration
- explore
- Explored
- Exploring
- extend
- facilitates
- Feature
- Features
- First
- follows
- For
- from
- function
- functionality
- given
- grasp
- guide
- handle
- helpful
- High
- How
- However
- HTTPS
- implement
- implementation
- implemented
- implementing
- in
- inheritance
- inner
- insights
- instance
- instances
- instead
- interested
- into
- Introduction
- IT
- ITS
- itself
- jpg
- Key
- Know
- language
- lastly
- later
- learned
- legs
- Length
- less
- lies
- Main
- make
- MAKES
- Making
- Manipulation
- max-width
- May..
- mechanisms
- method
- methods
- mind
- mistakes
- modify
- more
- more efficient
- name
- Named
- Need
- no
- number
- of
- Offers
- on
- ONE
- operate
- or
- Organized
- our
- own
- parameter
- parameters
- particularly
- pass
- passed
- Passing
- plato
- Plato Data Intelligence
- PlatoData
- practices
- primary
- Programming
- proper
- provide
- provides
- purpose
- Python
- rather
- readers
- recommended
- refer
- refers
- represents
- required
- return
- returns
- same
- scenarios
- seeking
- SELF
- several
- side
- skills
- some
- square
- standard
- such
- suitable
- syntax
- than
- that
- The
- The Area
- Them
- There.
- These
- they
- this
- those
- Through
- to
- tools
- track
- typical
- typically
- understand
- understanding
- us
- Usage
- use
- used
- useful
- using
- usually
- utilize
- Utilizing
- Valuable
- value
- various
- versatile
- via
- vice
- want
- Way..
- we
- What
- What is
- when
- whereas
- which
- while
- will
- with
- within
- without
- Work
- working
- workings
- Yield
- Your
- zephyrnet