Introduction
A Linked List is a data structure consisting of a sequence of nodes, each containing a value and a reference to the next node in the sequence. Unlike arrays, Linked Lists do not require contiguous memory allocation, making them more flexible and efficient for certain operations. In this article, we will explore the advantages and disadvantages of Linked Lists and how to implement them in Python.
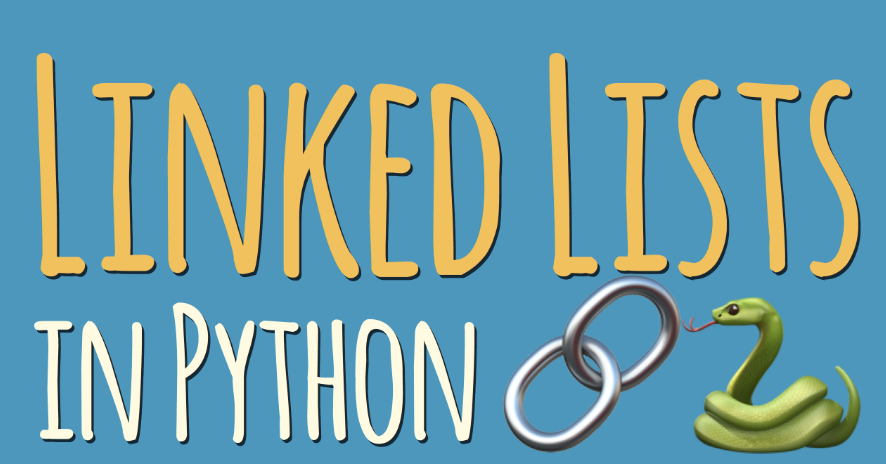
Table of contents
Advantages and Disadvantages of Linked Lists
Linked Lists offer several advantages over other data structures. Firstly, they allow for efficient insertion and deletion of elements, as they only require updating the references of neighboring nodes. This makes LinkedLists ideal for scenarios where frequent modifications are expected. Additionally, LinkedLists can dynamically grow or shrink in size, unlike arrays, which have a fixed size.
However, Linked Lists also have some disadvantages. Unlike arrays, Linked Lists do not support random access to elements, meaning that accessing an element at a specific index requires traversing the list from the beginning. This can result in slower performance for certain operations. Furthermore, Linked Lists require extra memory to store the references to the next nodes, which can be inefficient for small datasets.
Implementing Linked Lists in Python
Python provides a flexible and intuitive way to implement Linked Lists. There are three main types of Linked Lists: Singly Linked List, Doubly Linked List, and Circular Linked List. Let’s explore each of them in detail.
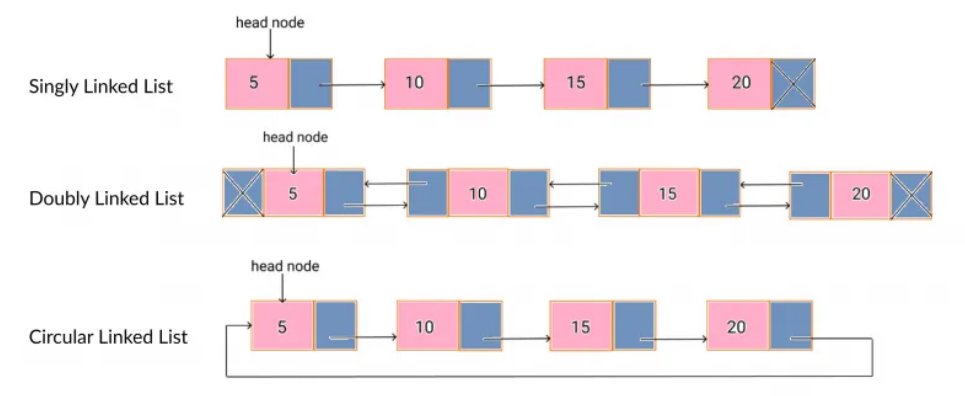
Singly Linked List
A Singly Linked List consists of nodes where each node contains a value and a reference to the next node in the sequence. Here’s how you can create a Singly Linked List in Python:
class Node:
def __init__(self, value):
self.value = value
self.next = None
class Linked List:
def __init__(self):
self.head = None
Creating a Singly Linked List
To create a Singly Linked List, we need to define a Node class that represents each node in the list. Each node contains a value and a reference to the next node. The Linked List class serves as the container for the nodes, with the head attribute pointing to the first node in the list.
Inserting Nodes in a Singly Linked List
Inserting nodes in a Singly Linked List involves updating the references of neighboring nodes. Here’s an example of inserting a node at the beginning of the list:
def insert_at_beginning(self, value):
new_node = Node(value)
new_node.next = self.head
self.head = new_node
Deleting Nodes from a Singly Linked List
Deleting nodes from a Singly Linked List requires updating the references of neighboring nodes. Here’s an example of deleting a node with a specific value:
def delete_node(self, value):
current = self.head
if current.value == value:
self.head = current.next
else:
while current.next:
if current.next.value == value:
current.next = current.next.next
break
current = current.next
Searching in a Singly Linked List
Searching for a specific value in a Singly Linked List involves traversing the list until the value is found or the end of the list is reached. Here’s an example of searching for a value:
def search(self, value):
current = self.head
while current:
if current.value == value:
return True
current = current.next
return False
Reversing a Singly Linked List
Reversing a Singly Linked List requires updating the references of each node to point to the previous node. Here’s an example of reversing a Singly Linked List:
def reverse(self):
previous = None
current = self.head
while current:
next_node = current.next
current.next = previous
previous = current
current = next_node
self.head = previous
Doubly Linked List
A Doubly Linked List is similar to a Singly Linked List, but each node contains a reference to both the next node and the previous node in the sequence. This allows for efficient traversal in both directions. Here’s how you can create a Doubly Linked List in Python:
class Node:
def __init__(self, value):
self.value = value
self.next = None
self.previous = None
class DoublyLinked List:
def __init__(self):
self.head = None
Creating a Doubly Linked List
To create a Doubly Linked List, we define a Node class that contains a value, a reference to the next node, and a reference to the previous node. The DoublyLinked List class serves as the container for the nodes, with the head attribute pointing to the first node in the list.
Inserting Nodes in a Doubly Linked List
Inserting nodes in a Doubly Linked List involves updating the references of neighboring nodes. Here’s an example of inserting a node at the beginning of the list:
def insert_at_beginning(self, value):
new_node = Node(value)
if self.head:
self.head.previous = new_node
new_node.next = self.head
self.head = new_node
Deleting Nodes from a Doubly Linked List
Deleting nodes from a Doubly Linked List requires updating the references of neighboring nodes. Here’s an example of deleting a node with a specific value:
def delete_node(self, value):
current = self.head
if current.value == value:
self.head = current.next
if self.head:
self.head.previous = None
else:
while current.next:
if current.next.value == value:
current.next = current.next.next
if current.next:
current.next.previous = current
break
current = current.next
Searching in a Doubly Linked List
Searching for a specific value in a Doubly Linked List involves traversing the list in either direction until the value is found or the end of the list is reached. Here’s an example of searching for a value:
def search(self, value):
current = self.head
while current:
if current.value == value:
return True
current = current.next
return False
Reversing a Doubly Linked List
Reversing a Doubly Linked List requires updating the references of each node to swap the next and previous pointers. Here’s an example of reversing a Doubly Linked List:
def reverse(self):
current = self.head
while current:
next_node = current.next
current.next = current.previous
current.previous = next_node
if not next_node:
self.head = current
current = next_node
Circular Linked List
A Circular Linked List is a variation of a Singly Linked List where the last node points back to the first node, creating a circular structure. This allows for efficient traversal from any node in the list. Here’s how you can create a Circular Linked List in Python:
class Node:
def __init__(self, value):
self.value = value
self.next = None
class CircularLinked List:
def __init__(self):
self.head = None
Creating a Circular Linked List
To create a Circular Linked List, we define a Node class that contains a value and a reference to the next node. The CircularLinked List class serves as the container for the nodes, with the head attribute pointing to the first node in the list. Additionally, the last node’s next reference is set to the head, creating a circular structure.
Inserting Nodes in a Circular Linked List
Inserting nodes in a Circular Linked List involves updating the references of neighboring nodes. Here’s an example of inserting a node at the beginning of the list:
def insert_at_beginning(self, value):
new_node = Node(value)
if not self.head:
self.head = new_node
new_node.next = self.head
else:
current = self.head
while current.next != self.head:
current = current.next
current.next = new_node
new_node.next = self.head
self.head = new_node
Deleting Nodes from a Circular Linked List
Deleting nodes from a Circular Linked List requires updating the references of neighboring nodes. Here’s an example of deleting a node with a specific value:
def delete_node(self, value):
if not self.head:
return
current = self.head
if current.value == value:
while current.next != self.head:
current = current.next
if current == self.head:
self.head = None
else:
current.next = self.head.next
self.head = self.head.next
else:
previous = None
while current.next != self.head:
previous = current
current = current.next
if current.value == value:
previous.next = current.next
break
Searching in a Circular Linked List
Searching for a specific value in a Circular Linked List involves traversing the list until the value is found or the entire list is traversed. Here’s an example of searching for a value:
def search(self, value):
if not self.head:
return False
current = self.head
while True:
if current.value == value:
return True
current = current.next
if current == self.head:
break
return False
Reversing a Circular Linked List
Reversing a Circular Linked List requires updating the references of each node to reverse the circular structure. Here’s an example of reversing a Circular Linked List:
def reverse(self):
if not self.head:
return
previous = None
current = self.head
next_node = current.next
while True:
current.next = previous
previous = current
current = next_node
next_node = next_node.next
if current == self.head:
break
self.head = previous
Common Operations on Linked Lists
Linked Lists support various common operations that can be performed on the elements. Let’s explore some of these operations:
Accessing Elements in a Linked List
To access elements in a Linked List, we can traverse the list starting from the head node and move to the next node until we reach the desired position. Here’s an example of accessing an element at a specific index:
def get_element(self, index):
current = self.head
count = 0
while current:
if count == index:
return current.value
count += 1
current = current.next
raise IndexError("Index out of range")
Modifying Elements in a Linked List
Modifying elements in a Linked List involves traversing the list to find the desired element and updating its value. Here’s an example of modifying an element at a specific index:
def modify_element(self, index, new_value):
current = self.head
count = 0
while current:
if count == index:
current.value = new_value
return
count += 1
current = current.next
raise IndexError("Index out of range")
Finding the Length of a Linked List
To find the length of a Linked List, we can traverse the list and count the number of nodes. Here’s an example of finding the length of a Linked List:
def get_length(self):
current = self.head
count = 0
while current:
count += 1
current = current.next
return count
Checking if a Linked List is Empty
To check if a Linked List is empty, we can simply check if the head node is None. Here’s an example of checking if a Linked List is empty:
def is_empty(self):
return self.head is None
Concatenating Linked Lists
To concatenate two Linked Lists, we can traverse the first list to find the last node and update its next reference to the head of the second list. Here’s an example of concatenating two Linked Lists:
def concatenate(self, other_list):
if not self.head:
self.head = other_list.head
else:
current = self.head
while current.next:
current = current.next
current.next = other_list.head
Linked List vs. Array
Linked Lists and arrays are both commonly used data structures, but they have different characteristics that make them suitable for different scenarios. Let’s compare Linked Lists and arrays in terms of memory efficiency, insertion and deletion efficiency, and random access efficiency.
Memory Efficiency
Linked Lists are more memory-efficient than arrays because they do not require contiguous memory allocation. Each node in a Linked List only needs to store the value and a reference to the next node, whereas arrays need to allocate memory for all elements, even if they are not used.
Insertion and Deletion Efficiency
Linked Lists excel in insertion and deletion operations, especially when elements are frequently added or removed from the middle of the list. Inserting or deleting an element in a Linked List only requires updating the references of neighboring nodes, whereas arrays may require shifting elements to accommodate the change.
Random Access Efficiency
Arrays provide efficient random access to elements based on their indices, as they allow direct memory addressing. In contrast, Linked Lists require traversing the list from the beginning to access an element at a specific index, resulting in slower performance for random access operations.
Choosing the Right Data Structure
The choice between Linked Lists and arrays depends on the specific requirements of the application. If frequent modifications and dynamic resizing are expected, Linked Lists is a better choice. On the other hand, if random access and memory efficiency are crucial, arrays are more suitable.
Linked List Applications
Now that we have a good understanding of linked lists and how they work, let’s explore some of the practical applications where linked lists can be used effectively.
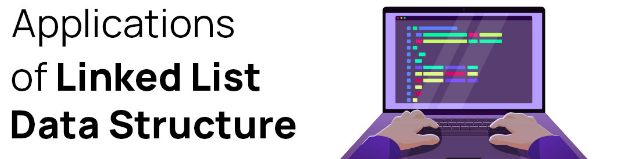
You can also enroll in our Free Courses Today!
Implementing Stacks and Queues
One of the most common applications of linked lists is implementing stacks and queues. Both stacks and queues are abstract data types that can be easily implemented using linked lists.
A stack is a data structure that follows the Last-In-First-Out (LIFO) principle. Elements are added and removed from the same end, known as the top of the stack. Linked lists provide an efficient way to implement stacks as we can easily add or remove elements from the head of the list.
Here’s an example of implementing a stack using a linked list in Python:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class Stack:
def __init__(self):
self.head = None
def push(self, data):
new_node = Node(data)
new_node.next = self.head
self.head = new_node
def pop(self):
if self.head is None:
return None
popped = self.head.data
self.head = self.head.next
return popped
A queue, on the other hand, follows the First-In-First-Out (FIFO) principle. Elements are added at one end, known as the rear, and removed from the other end, known as the front. Linked lists can also be used to implement queues efficiently.
Here’s an example of implementing a queue using a linked list in Python:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class Queue:
def __init__(self):
self.front = None
self.rear = None
def enqueue(self, data):
new_node = Node(data)
if self.rear is None:
self.front = new_node
self.rear = new_node
else:
self.rear.next = new_node
self.rear = new_node
def dequeue(self):
if self.front is None:
return None
dequeued = self.front.data
self.front = self.front.next
if self.front is None:
self.rear = None
return dequeued
Handling Large Datasets
Linked lists are also useful when dealing with large datasets. Unlike arrays, linked lists do not require contiguous memory allocation. This means that linked lists can efficiently handle datasets of varying sizes without the need for resizing or reallocation.
For example, let’s say we have a dataset of millions of records, and we need to perform operations such as insertion, deletion, or traversal. Using an array for this task can be inefficient as it requires shifting elements when inserting or deleting. However, with a linked list, we can easily insert or delete elements by updating the pointers, resulting in faster operations.
Graph Traversal Algorithms
Graph traversal algorithms, such as breadth-first search (BFS) and depth-first search (DFS), can also be implemented using linked lists. In graph traversal, we visit each vertex or node in a graph in a specific order.
Linked lists can be used to represent the adjacency list of a graph, where each node in the linked list represents a vertex and its adjacent vertices are stored as linked list nodes. This representation allows for efficient traversal and exploration of the graph.
Polynomial Representation
Linked lists can be used to represent polynomials efficiently. In mathematics, polynomials are expressions consisting of variables and coefficients. Each term in a polynomial can be represented as a node in a linked list, where the coefficient and exponent are stored as data.
By using linked lists, we can easily perform operations on polynomials, such as addition, subtraction, and multiplication. The nodes can be manipulated to perform these operations, resulting in a concise and efficient representation of polynomials.
Music and Video Playlists
Linked lists are commonly used to implement playlists in music and video players. Each song or video can be represented as a node in a linked list, where the data contains information about the media file and the pointer points to the next song or video in the playlist.
Using linked lists for playlists allows for easy navigation between songs or videos. We can easily add or remove songs from the playlist by updating the pointers, providing a seamless user experience.
Conclusion
In conclusion, linked lists are versatile data structures that find applications in various domains. They can be used to implement stacks, and queues, handle large datasets, perform graph traversal, represent polynomials, and create playlists. Linked lists provide efficient solutions to these problems by leveraging their dynamic memory allocation and easy manipulation of nodes.
By understanding the applications of linked lists, we can make informed decisions when choosing data structures for our programs. Whether it’s managing data, implementing algorithms, or creating user-friendly interfaces, linked lists offer a valuable tool in the programmer’s toolkit.
So, the next time you encounter a problem that requires efficient insertion, deletion, or traversal, consider using linked lists to simplify your solution and optimize your code.
You can also read more articles related to Python Lists here:
Frequently Asked Questions
A: A Linked List is a data structure consisting of nodes, where each node contains a value and a reference (or link) to the next node in the sequence.
A: Linked Lists offer efficient insertion and deletion operations, dynamic resizing, and do not require contiguous memory allocation.
A: Linked Lists lack random access, requiring traversal for element access. They also consume extra memory for storing references, which may be inefficient for small datasets.
A: The main types of Linked Lists are Singly Linked List, Doubly Linked List, and Circular Linked List.
A: Linked Lists are more memory-efficient than arrays when dealing with dynamic resizing and frequent insertions or deletions, as they do not require contiguous memory allocation.
Related
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/02/linked-lists-in-python/
- :is
- :not
- :where
- 1
- 10
- 16
- 48
- 5
- 9
- a
- About
- ABSTRACT
- access
- accessing
- accommodate
- add
- added
- addition
- Additionally
- addressing
- adjacent
- advantages
- algorithms
- All
- allocate
- allocation
- allow
- allows
- also
- an
- and
- any
- Application
- applications
- ARE
- Array
- article
- articles
- AS
- At
- back
- based
- BE
- because
- Beginning
- Better
- between
- both
- Break
- but
- by
- CAN
- certain
- change
- characteristics
- check
- checking
- choice
- choosing
- circular
- class
- code
- coefficients
- Common
- commonly
- compare
- concise
- conclusion
- Consider
- Consisting
- consists
- consume
- Container
- contains
- contrast
- count
- create
- Creating
- crucial
- Current
- data
- datasets
- dealing
- decisions
- def
- define
- delete
- depends
- desired
- detail
- different
- direct
- direction
- directions
- do
- domains
- doubly
- dynamic
- dynamically
- each
- easily
- easy
- effectively
- efficiency
- efficient
- efficiently
- either
- element
- elements
- else
- empty
- encounter
- end
- enroll
- Entire
- especially
- Ether (ETH)
- Even
- example
- Excel
- expected
- experience
- exploration
- explore
- exponent
- expressions
- extra
- false
- faster
- File
- Find
- finding
- First
- fixed
- flexible
- follows
- For
- found
- frequent
- frequently
- from
- front
- Furthermore
- good
- graph
- Grow
- hand
- handle
- Have
- head
- here
- High
- How
- How To
- However
- HTTPS
- ideal
- if
- implement
- implemented
- implementing
- in
- index
- Indices
- inefficient
- information
- informed
- interfaces
- intuitive
- involves
- IT
- ITS
- known
- Lack
- large
- Last
- Length
- leveraging
- LINK
- linked
- List
- Lists
- Main
- make
- MAKES
- Making
- managing
- manipulated
- Manipulation
- mathematics
- max-width
- May..
- meaning
- means
- Media
- Memory
- Middle
- millions
- Modifications
- more
- most
- move
- multiplication
- Music
- Navigation
- Need
- needs
- neighboring
- next
- node
- nodes
- None
- number
- of
- offer
- on
- ONE
- only
- Operations
- Optimize
- or
- order
- Other
- our
- out
- over
- perform
- performance
- performed
- plato
- Plato Data Intelligence
- PlatoData
- players
- Point
- points
- polynomial
- polynomials
- position
- Practical
- Practical Applications
- previous
- principle
- Problem
- problems
- Programs
- provide
- provides
- providing
- Python
- raise
- random
- range
- reach
- reached
- Read
- records
- reference
- references
- related
- remove
- Removed
- represent
- representation
- represented
- represents
- require
- Requirements
- requires
- result
- resulting
- return
- reverse
- right
- same
- say
- scenarios
- seamless
- Search
- searching
- Second
- SELF
- Sequence
- serves
- set
- several
- SHIFTING
- similar
- simplify
- simply
- Size
- sizes
- small
- solution
- Solutions
- some
- song
- specific
- stack
- Stacks
- Starting
- store
- stored
- structure
- structures
- subtraction
- such
- suitable
- support
- SVG
- swap
- Task
- term
- terms
- than
- that
- The
- The Graph
- their
- Them
- There.
- These
- they
- this
- three
- time
- to
- tool
- toolkit
- top
- traverse
- true
- two
- type
- types
- understanding
- unlike
- until
- Update
- updating
- used
- useful
- User
- User Experience
- user-friendly
- using
- Valuable
- value
- variables
- various
- varying
- versatile
- Video
- Videos
- Visit
- vs
- Way..
- we
- What
- What is
- when
- whereas
- whether
- which
- while
- will
- with
- without
- Work
- you
- Your
- zephyrnet