Introduction
AI agent development is one of the hottest frontiers of Software innovation. As the quality of Large Language Models evolves, we will witness a surge in AI agent integration with existing software systems. With AI agents, it will be possible to accomplish tasks with voice or gesture commands instead of manually navigating through applications. But right now, agent development is in its nascent stage. We are still going through the initial phase of infrastructure, tools, and framework development, similar to the Internet of the 1990s. So, in this article, we will discuss another framework for agent development called CrewAI.
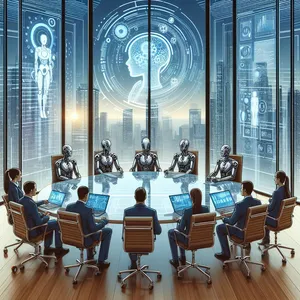
Learning Objectives
- Learn about AI agents.
- Explore CrewAI – an open-source tool for building agents.
- Build a collaborative AI crew for writing content.
- Explore real-life use cases of AI agents.
This article was published as a part of the Data Science Blogathon.
Table of contents
What are AI Agents?
The language models excel at translation, summarizing, and reasoning. However, you can do so much with them. One of the ways to fully realize the reasoning potential is to make LLMs agentic. The AI agents are LLMs augmented with the right tools and prompts. These agents can automate browsing, web scrapping, SQL query execution, file operations, and more. The agents use the reasoning capacity of LLMs to select a tool based on current requirements. But instead of using a single agent for a task, we can ensemble many of them to accomplish complex tasks.
Langchain is the default tool that comes to mind when discussing AI agents. However, manually orchestrating AI agents to perform collaborative tasks would be challenging with Langchain. This is where CrewAI comes into the picture.
What is CrewAI?
CrewAI is an open-source framework for orchestrating role-playing and autonomous AI agents. It helps create collaborative AI agents to accomplish complex goals with ease. The framework is designed to enable AI agents to assume roles, delegate tasks, and share goals, much like a real-world crew. These are some of the unique features of the CrewAI:
- Role-based Agents: We can define agents with specific roles, goals, and backstories to give more context to LLMs before answer generation.
- Task management: Define tasks with tools and dynamically assign them to agents.
- Inter-agent delegation: The agents can delegate tasks to other agents to collaborate effectively.
Below is a representation of the CrewAI mind map.
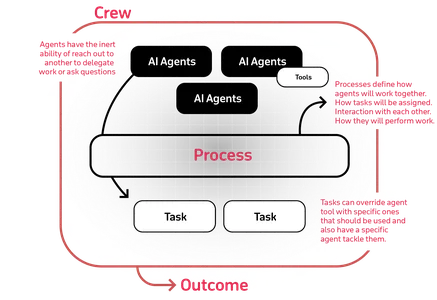
The CrewAI seamlessly integrates with the Langchain ecosystem. This means we can use the Langchain tools and LLM integrations with CrewAI.
Building a Collaborative AI Crew
To understand CrewAI better, let’s build collaborative AI agents for creative content writing. For this, we will define agents, tools, and the respective tasks for each agent. As it is a team for content writing, we will define three separate agents, like an idea analyst, a writer, and an editor. Each agent will be assigned a task.
The analyst agent will be responsible for analyzing the idea and preparing a comprehensive blueprint for writing the content. The Writer agent will prepare the draft for the article, and finally, the editor will be responsible for formatting, editing, and correcting the draft. As we know, CrewAI lets us augment agents with custom tools. We will augment the editor with a tool to save it to the local disk. But to accomplish all these things, we need an LLM. Here, we will use Google’s Gemini model.
Let’s delve into the coding
As with any Python project, create a virtual environment and install the dependencies. We will need the Crewai library and Langchain’s implementation of Google GenAI. You can use other LLMs, like open-access models from Together, Any scale, or OpenAI models.
pip install crewai langchain-google-genai
The next step is to define our LLM and collaborative Agents. Create a separate file named agents.py to define agents.
import os
from crewai import Agent
from langchain.tools import tool
from langchain_google_genai import GoogleGenerativeAI
GOOGLE_API_KEY = "Your Key"
llm = GoogleGenerativeAI(
model="gemini-pro",
google_api_key=GOOGLE_API_KEY
)
Let’s define the file-saving tool.
class FileTools:
@tool("Write File with content")
def write_file(data: str):
"""Useful to write a file to a given path with a given content.
The input to this tool should be a pipe (|) separated text
of length two, representing the full path of the file,
including the ./lore/, and the written content you want to write to it.
"""
try:
path, content = data.split("|")
path = path.replace("n", "").replace(" ", "").replace("`", "")
if not path.startswith("./lore"):
path = f"./lore/{path}"
with open(path, "w") as f:
f.write(content)
return f"File written to {path}."
except Exception:
return "Error with the input format for the tool."
The above write_file method is decorated with Langchain’s tool function. As the CrewAI uses Langchain under the hood, the tools must comply with Langchain’s conventions. The function expects a single string with two parts, a file path, and content separated by a pipe (|). The method doc strings are also used as added context for the function. So, make sure you give detailed information about the method.
Let’s define the agents
idea_analyst = Agent(
role = "Idea Analyst",
goal = "Comprehensively analyse an idea to prepare blueprints for the article to be written",
backstory="""You are an experienced content analyst, well versed in analyzing
an idea and preparing a blueprint for it.""",
llm = llm,
verbose=True
)
writer = Agent(
role = "Fiction Writer",
goal = "Write compelling fantasy and sci-fi fictions from the ideas given by the analyst",
backstory="""A renowned fiction-writer with 2 times NYT
a best-selling author in the fiction and sci-fi category.""",
llm=llm,
verbose=True
)
editor = Agent(
role= "Content Editor",
goal = "Edit contents written by writer",
backstory="""You are an experienced editor with years of
experience in editing books and stories.""",
llm = llm,
tools=[FileTools.write_file],
verbose=True
)
We have three agents, each with a different role, goal, and backstory. This information is used as a prompt for the LLM to give more context. The editor agent has a writing tool associated with it.
The next thing is to define tasks. For this, create a different file tasks.py.
from textwrap import dedent
class CreateTasks:
def expand_idea():
return dedent(""" Analyse the given task {idea}. Prepare comprehensive pin-points
for accomplishing the given task.
Make sure the ideas are to the point, coherent, and compelling.
Make sure you abide by the rules. Don't use any tools.
RULES:
- Write ideas in bullet points.
- Avoid adult ideas.
""")
def write():
return dedent("""Write a compelling story in 1200 words based on the blueprint
ideas given by the Idea
analyst.
Make sure the contents are coherent, easily communicable, and captivating.
Don't use any tools.
Make sure you abide by the rules.
RULES:
- Writing must be grammatically correct.
- Use as little jargon as possible
""")
def edit():
return dedent("""
Look for any grammatical mistakes, edit, and format if needed.
Add title and subtitles to the text when needed.
Do not shorten the content or add comments.
Create a suitable filename for the content with the .txt extension.
You MUST use the tool to save it to the path ./lore/(your title.txt).
""")
The tasks here are detailed action plans you expect the agents to perform.
Finally, create the main.py file where we assemble the Agents and Tasks to create a functional crew.
from textwrap import dedent
from crewai import Crew, Task
from agents import editor, idea_analyst, writer
from tasks import CreateTasks
class ContentWritingCrew():
def __init__(self, idea):
self.idea = idea
def __call__(self):
tasks = self._create_tasks()
crew = Crew(
tasks=tasks,
agents=[idea_analyst, writer, editor],
verbose=True
)
result = crew.kickoff()
return result
def _create_tasks(self):
idea = CreateTasks.expand_idea().format(idea=self.idea)
expand_idea_task = Task(
description=idea,
agent = idea_analyst
)
write_task = Task(
description=CreateTasks.write(),
agent=writer
)
edit_task = Task(
description=CreateTasks.edit(),
agent=editor
)
return [expand_idea_task, write_task, edit_task]
if __name__ == "__main__":
dir = "./lore"
if not os.path.exists(dir):
os.mkdir(dir)
idea = input("idea: ")
my_crew = ContentWritingCrew(idea=idea)
result = my_crew()
print(dedent(result))
In the above code, we defined a ContentWritingCrew class that accepts an idea string from the user. The _create_tasks method creates tasks. The __call__ method initializes and kicks off the crew. Once you run the script, you can observe the chain of actions on the terminal or notebook. The tasks will be executed in the order they are defined by the crew. Here is a snapshot of the execution log.
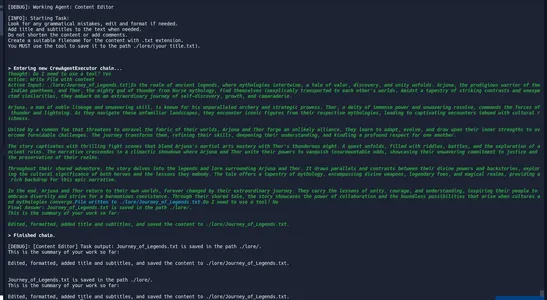
This is the execution log for the final agent. i.e. Editor. It edits the draft received from the writer’s agent and uses the file-writing tool to save the file with a suitable filename.
This is the general workflow for creating collaborative AI agents with CrewAI. You can pair other Langchain tools or create custom tools with efficient prompting to accomplish more complex tasks.
Here is the GitHub repository for the codes: sunilkumardash9/ContentWritingAgents.
Replit repository: Sunil-KumarKu17/CollborativeAIAgent
Real-world Use Cases
Autonomous AI agents can have a lot of use cases. From personal assistants to virtual instructors. Here are a few use cases of AI agents.
- Personal AI Assistant: Personal Assistants will be an integral part of us soon. A Jarvis-like assistant that processes all your data provides insight as you go and handles trivial tasks by itself.
- Code interpreters: OpenAI’s code interpreter is a brilliant example of an AI agent. The interpreter can run any Python script and output the results in response to a text prompt. This is arguably the most successful agent to date.
- Virtual Instructors: As the AI tech evolves, we can expect virtual instructors in many fields like education, training, etc.
- Agent First Software: A huge potential use case of AI agents is in agent first software development. Instead of manually browsing and clicking buttons to get things done, AI agents will automatically accomplish them based on voice commands.
- Spatial Computing: As the AR/VR tech evolves, AI agents will play a crucial role in bridging the gap between the virtual and real world.
Conclusion
We are still in the early stages of AI agent development. Currently, for the best possible outcome from AI agents, we need to rely on GPT-4, and it is expensive. But as the open-source models catch up to GPT-4, we will get better options for running AI agents efficiently at a reasonable cost. On the other hand, the frameworks for agent development are progressing rapidly. As we move forward, the frameworks will enable agents to perform even more complex tasks.
Key Takeaways
- AI agents leverage the reasoning capacity of LLMs to select appropriate tools to accomplish complex tasks.
- CrewAI is an open-source framework for building collaborative AI agents.
- The unique feature of CrewAI includes role-based Agents, autonomous inter-agent delegation, and flexible task management.
- CrewAI seamlessly integrates with the existing Langchain ecosystem. We can use Langchain tools and LLM integrations with CrewAI.
Frequently Asked Questions
A. AI agents are software programs that interact with their environment, make decisions, and act to achieve an end goal.
A. This depends on your use cases and budget. GPT 4 is the most capable but expensive, while GPT 3.5, Mixtral, and Gemini Pro models are less qualified but fast and cheap.
A. CrewAI is an open-source framework for orchestrating role-playing and autonomous AI agents. It helps create collaborative AI agents to accomplish complex goals with ease.
A. CrewAI provides a high-level abstraction for building collaborative AI agents for complex workflows.
A. In Autogen, orchestrating agents’ interactions requires additional programming, which can become complex and cumbersome as the scale of tasks grows.
The media shown in this article is not owned by Analytics Vidhya and is used at the Author’s discretion.
Related
- SEO Powered Content & PR Distribution. Get Amplified Today.
- PlatoData.Network Vertical Generative Ai. Empower Yourself. Access Here.
- PlatoAiStream. Web3 Intelligence. Knowledge Amplified. Access Here.
- PlatoESG. Carbon, CleanTech, Energy, Environment, Solar, Waste Management. Access Here.
- PlatoHealth. Biotech and Clinical Trials Intelligence. Access Here.
- Source: https://www.analyticsvidhya.com/blog/2024/01/building-collaborative-ai-agents-with-crewai/
- :has
- :is
- :not
- :where
- $UP
- 10
- 11
- 16
- 8
- a
- About
- above
- abstraction
- Accepts
- accomplish
- accomplishing
- Achieve
- Act
- Action
- actions
- add
- added
- Additional
- Adult
- Agent
- agents
- AI
- All
- also
- an
- analyse
- analyst
- analytics
- Analytics Vidhya
- analyzing
- and
- Another
- answer
- any
- applications
- appropriate
- AR/VR
- ARE
- arguably
- article
- AS
- assigned
- Assistant
- assistants
- associated
- assume
- At
- augment
- augmented
- author
- automate
- automatically
- autonomous
- avoid
- based
- BE
- become
- before
- BEST
- Better
- between
- blogathon
- blueprint
- Books
- bridging
- brilliant
- Browsing
- budget
- build
- Building
- but
- buttons
- by
- called
- CAN
- capable
- Capacity
- captivating
- case
- cases
- Catch
- Category
- chain
- challenging
- cheap
- class
- code
- codes
- COHERENT
- collaborate
- collaborative
- comes
- comments
- compelling
- compelling story
- complex
- comply
- comprehensive
- content
- Content Writing
- contents
- context
- Conventions
- correct
- Cost
- create
- creates
- Creating
- Creative
- crew
- crucial
- cumbersome
- Current
- Currently
- custom
- Dash
- data
- Date
- decisions
- Default
- define
- defined
- delegation
- delve
- dependencies
- depends
- designed
- detailed
- Development
- difference
- different
- discretion
- discuss
- discussing
- do
- don
- done
- draft
- dynamically
- e
- each
- Early
- ease
- easily
- ecosystem
- editing
- editor
- Education
- effectively
- efficient
- efficiently
- enable
- end
- Environment
- error
- etc
- Ether (ETH)
- Even
- evolves
- example
- Excel
- Except
- exception
- executed
- execution
- existing
- expect
- expects
- expensive
- experience
- experienced
- extension
- FANTASY
- FAST
- Feature
- Features
- few
- Fiction
- Fields
- File
- final
- Finally
- First
- flexible
- For
- format
- Forward
- Framework
- frameworks
- from
- Frontiers
- full
- fully
- function
- functional
- gap
- Gemini
- General
- generation
- gesture
- get
- GitHub
- Give
- given
- Go
- goal
- Goals
- going
- Google’s
- Grows
- hand
- Handles
- Have
- helps
- here
- high-level
- hood
- hottest
- However
- HTTPS
- huge
- i
- idea
- ideas
- if
- implementation
- import
- in
- includes
- Including
- information
- Infrastructure
- initial
- Innovation
- input
- insight
- install
- instead
- integral
- Integrates
- integration
- integrations
- interact
- interactions
- Internet
- into
- IT
- ITS
- itself
- jargon
- jpg
- Key
- Kicks
- Know
- kumar
- language
- large
- Length
- less
- Lets
- Leverage
- Library
- like
- little
- local
- log
- Look
- Lot
- Main
- make
- management
- manually
- many
- map
- means
- Media
- method
- mind
- mistakes
- model
- models
- more
- most
- move
- move forward
- much
- must
- Named
- nascent
- navigating
- Need
- needed
- next
- notebook
- now
- NYT
- observe
- of
- off
- on
- once
- ONE
- open source
- OpenAI
- Operations
- Options
- or
- orchestrating
- order
- OS
- Other
- our
- Outcome
- output
- owned
- pair
- part
- parts
- path
- perform
- personal
- phase
- picture
- pipe
- plans
- plato
- Plato Data Intelligence
- PlatoData
- Play
- Point
- points
- possible
- potential
- Prepare
- preparing
- Pro
- processes
- Programming
- Programs
- progressing
- project
- prompts
- provides
- published
- Python
- qualified
- quality
- rapidly
- real
- real world
- realize
- reasonable
- received
- rely
- Renowned
- repository
- representation
- representing
- Requirements
- requires
- respective
- response
- responsible
- result
- Results
- return
- right
- Role
- Role-Playing
- roles
- rules
- Run
- running
- Save
- Scale
- sci-fi
- Science
- script
- seamlessly
- select
- SELF
- separate
- Share
- should
- shown
- similar
- single
- Snapshot
- So
- Software
- software development
- some
- Soon
- specific
- SQL
- Stage
- stages
- Step
- Still
- Stories
- Story
- String
- subtitles
- successful
- suitable
- sure
- surge
- Systems
- T
- Task
- tasks
- team
- tech
- Terminal
- text
- that
- The
- their
- Them
- These
- they
- thing
- things
- this
- three
- Through
- times
- Title
- to
- tool
- tools
- Training
- Translation
- try
- two
- under
- understand
- unique
- unique features
- us
- use
- use case
- Use Cases Of AI
- used
- useful
- User
- uses
- using
- versed
- Virtual
- Voice
- voice commands
- W
- want
- was
- ways
- we
- web
- webp
- WELL
- What
- What is
- when
- which
- while
- will
- with
- witness
- words
- workflow
- workflows
- world
- would
- write
- writer
- writing
- written
- years
- you
- Your
- zephyrnet